Section 4.9 Built-In Functions
The built-in functions of MATLAB can operate on individual elements or on all elements of an array at once. For example, the cosine function
cos()
can be used to find the cosine of a whole bunch of numbers (arranged as a vector, for example) all at once. Take a look:>> x = [0 pi/4 pi/2 3*pi/4 pi]; >> y = cos(x) >>
y = 1.0000 0.7071 0.0000 -0.7071 -1.0000
The result of calling such a function with an array as argument is another array of the same size as the argument. Here is why this might be useful:
>> x = 0:pi/24:3*pi; % 73 steps from 0 to 3pi >> y = cos(0.1*x).*cos(x); % the result is a 73-element vector >> plot(x,y)
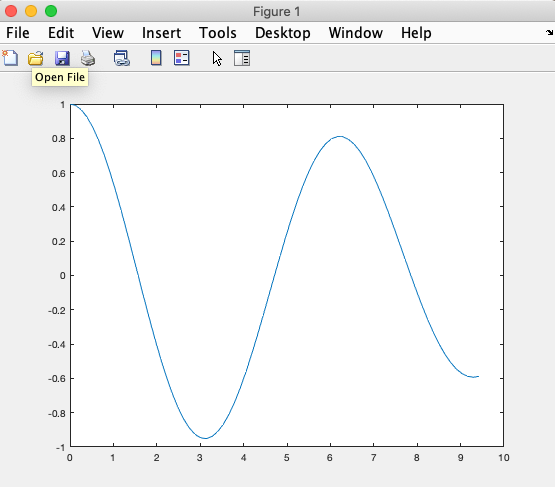
In C you would have had to write a loop in order to individually evaluate the cosine function on all desired x-values as is demonstrated in the following code snippet:
#define N 73
#define PI 3.14159265
float x[N], y[N];
for (i=0;i<=73;i++) {
x[i] = i*PI/24;
y[i] = cos(0.1*x[i]) * cos(x[i]);
}
This feature of MATLAB, in which functions accept arrays as arguments and return arrays with the same dimensions and size is called vectorization. We will see later that you can write a for-loop just as in C, but it is significantly slower to execute than the vectorized equivalent. Use vectorization whenever possible!
Subsection 4.9.1 Vector Functions
In addition to many MATLAB functions being vectorized, there are functions that operate on vectors and return a result that is not computed element-by-element but rather a function of the entire vector. Here are some examples:
mean(v)
: returns the mean (average) of the elements in the vector vmedian(v)
: returns the median of the elements in the vector vstd(v)
: returns the standard deviation of the elements in the vector vsum(v)
: returns the sum of the elements of the vector vsort(v)
: returns a new vector whose elements are those of v, arranged in ascending orderSome MATLAB functions can be called in multiple ways and will behave slightly differently depending on the call. For example:
d = max(v)
: returns the largest element of the vector v (and stores this in d)[d,n] = max(v)
: returns both the largest element of the vector v (and stores this in d) as well as the index (location) of this element (which is then stored in the variable n)d = min(v)
: returns the smallest element of the vector v[d,n] = min(v)
: returns the smallest element as well as its indexSubsection 4.9.2 Matrix Functions
When you apply a vector function on an entire matrix, the function will operate on each column of the matrix (as if this column were a vector entry). Here are some examples, assuming that A is a matrix:
d = max(A)
: returns a row vector containing the maximum element of each column of A[d,n] = max(A)
: returns two row vectors d and n, where d contains the largest elements and n their indicesHere is an example:
>> A = [1 2 3; 4 5 6; -5 3 20]; >> [d n] = max(A) >>
d = 4 5 20
n = 2 2 3
The
min
function works similarly:d = min(A)
: row vector containing minimum elements of A[d,n] = min(A)
: two row vectors containing smallest elements and indicesHere are some further functions that operate on arrays:
det(A)
: returns determinant of the matrix A (a number)inv(A)
: returns the inverse of the invertible square matrix A (a matrix)Also useful in linear algebra are the following functions:
dot(a,b)
: returns the scalar product of two vectors a and b (works on row or column vectors), the result is a numbercross(a,b)
: returns the cross product of two vectors, which each must have 3 elements, the result is a 3-element vectorSubsection 4.9.3 Random Number Functions
Certain simulations in science and engineering require random numbers. MATLAB provides several functions that calculate random numbers.
rand
: single random number between 0 and 1rand(1,n)
: n-element row vector of random numbers from the interval [0,1]rand(n)
: n×n matrix of random numbers from the interval [0,1]rand(m,n)
: m×n matrix of random numbers from the interval [0,1]randperm(n)
: n-element row vector with a random permutation of the integers 1 to nHere is an example:
>> randperm(6)
ans =
5 2 3 1 4 6
Often you need random numbers distributed uniformly throughout an interval that is not 0 to 1, but a to b. This can be accomplished by using:
a + (b-a) * rand(1,n)
>> a = 50; b = 100; n = 20;
>> a + (b-a) * rand(1,n)
This will produce 20 uniformly distributed random numbers between 50 and 100, outputted into the default ans variable.
Sometimes you need normally distributed random numbers; in this case use
randn
instead. It has the same formats as rand
but generates normally distributed numbers with a mean of 0 and standard deviation of 1. One can change both the mean and standard deviation as demonstrated here:(stdv * randn(1,n)) + mean
>> scores = round(13*randn(1,50)+75);
This generates 50 random integers with a mean of 75 and a standard deviation of 13.